与 Keras 进行面部检测和识别
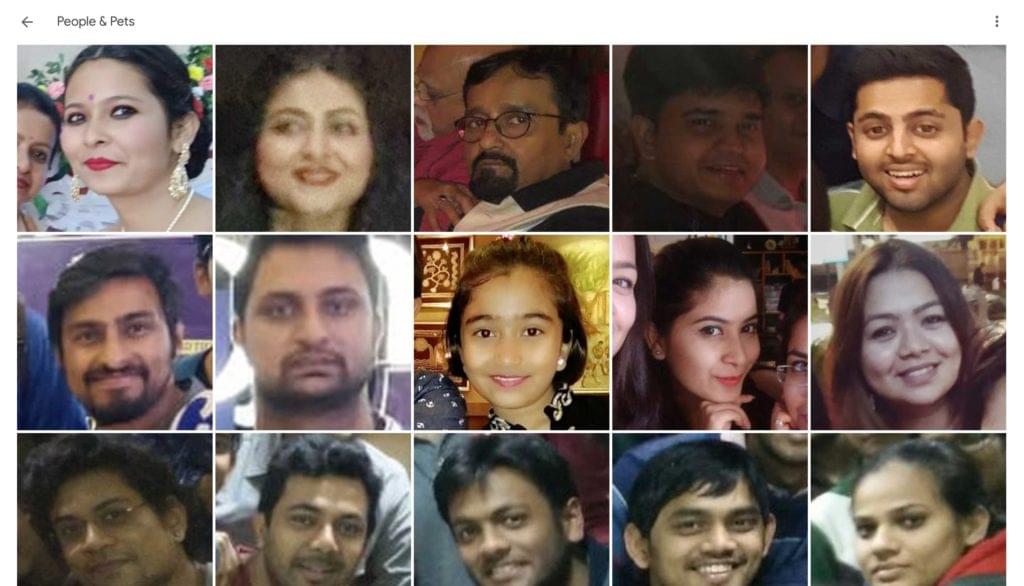
先决条件
matplotlib
pip3 install matplotlib
keras
pip3 install keras
pip
pip3 install mtcnn
pip3 install keras_vggface
第一步:利用MTCNN模型进行人脸检测
检索本地服务器外部托管的映像 通读图像 matplotlib
的 读入() 功能 通过MTCNN算法检测和探索人脸 从图像中提取面
1.1存储外部图像
store_image
import urllib.request
def store_image(url, local_file_name):
with urllib.request.urlopen(url) as resource:
with open(local_file_name, 'wb') as f:
f.write(resource.read())
store_image('https://ichef.bbci.co.uk/news/320/cpsprodpb/5944/production/_107725822_55fd57ad-c509-4335-a7d2-bcc86e32be72.jpg',
'iacocca_1.jpg')
store_image('https://www.gannett-cdn.com/presto/2019/07/03/PDTN/205798e7-9555-4245-99e1-fd300c50ce85-AP_080910055617.jpg?width=540&height=&fit=bounds&auto=webp',
'iacocca_2.jpg')
1.2检测图像中的人脸
matplotlib
from matplotlib import pyplot as plt
from mtcnn.mtcnn import MTCNN
imread()
image = plt.imread('iacocca_1.jpg')
MTCNN()
.detect_faces()
detector = MTCNN()
faces = detector.detect_faces(image)
for face in faces:
print(face)
box
keypoints
{'box': [160, 40, 35, 44], 'confidence': 0.9999798536300659, 'keypoints': {'left_eye': (172, 57), 'right_eye': (188, 57), 'nose': (182, 64), 'mouth_left': (173, 73), 'mouth_right': (187, 73)}}
1.3突出显示图像中的面
Rectangle
from matplotlib.patches import Rectangle
highlight_faces
imshow()
.show()
def highlight_faces(image_path, faces):
# display image
image = plt.imread(image_path)
plt.imshow(image)
ax = plt.gca()
# for each face, draw a rectangle based on coordinates
for face in faces:
x, y, width, height = face['box']
face_border = Rectangle((x, y), width, height,
fill=False, color='red')
ax.add_patch(face_border)
plt.show()
highlight_faces()
highlight_faces('iacocca_1.jpg', faces)
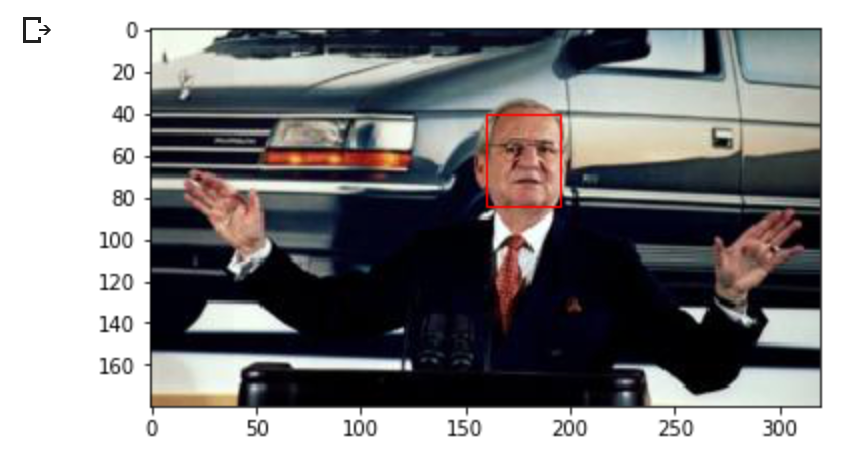
image = plt.imread('iacocca_2.jpg')
faces = detector.detect_faces(image)
highlight_faces('iacocca_2.jpg', faces)
1.4提取人脸进行进一步分析
extract_face_from_image()
from numpy import asarray
from PIL import Image
def extract_face_from_image(image_path, required_size=(224, 224)):
# load image and detect faces
image = plt.imread(image_path)
detector = MTCNN()
faces = detector.detect_faces(image)
face_images = []
for face in faces:
# extract the bounding box from the requested face
x1, y1, width, height = face['box']
x2, y2 = x1 + width, y1 + height
# extract the face
face_boundary = image[y1:y2, x1:x2]
# resize pixels to the model size
face_image = Image.fromarray(face_boundary)
face_image = face_image.resize(required_size)
face_array = asarray(face_image)
face_images.append(face_array)
return face_images
extracted_face = extract_face_from_image('iacocca_1.jpg')
# Display the first face from the extracted faces
plt.imshow(extracted_face[0])
plt.show()
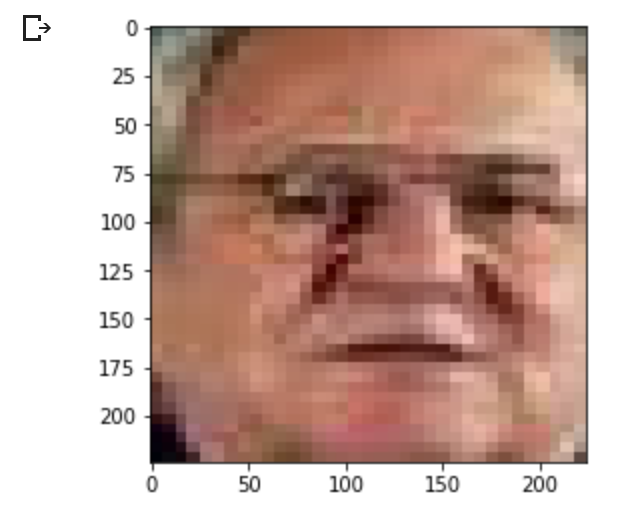
第二步:利用VGGFace2模型进行人脸识别
2.1比较两个面
VGGFace
from keras_vggface.utils import preprocess_input
from keras_vggface.vggface import VGGFace
from scipy.spatial.distance import cosine
def get_model_scores(faces):
samples = asarray(faces, 'float32')
# prepare the data for the model
samples = preprocess_input(samples, version=2)
# create a vggface model object
model = VGGFace(model='resnet50',
include_top=False,
input_shape=(224, 224, 3),
pooling='avg')
# perform prediction
return model.predict(samples)
faces = [extract_face_from_image(image_path)
for image_path in ['iacocca_1.jpg', 'iacocca_2.jpg']]
model_scores = get_model_scores(faces)
cosine()
if cosine(model_scores[0], model_scores[1]) <= 0.4:
print("Faces Matched")
Faces Matched
2.2比较两个图像中的多个面
store_image('https://cdn.vox-cdn.com/thumbor/Ua2BXGAhneJHLQmLvj-ZzILK-Xs=/0x0:4872x3160/1820x1213/filters:focal(1877x860:2655x1638):format(webp)/cdn.vox-cdn.com/uploads/chorus_image/image/63613936/1143553317.jpg.5.jpg',
'chelsea_1.jpg')
image = plt.imread('chelsea_1.jpg')
faces_staring_xi = detector.detect_faces(image)
highlight_faces('chelsea_1.jpg', faces_staring_xi)
store_image('https://cdn.vox-cdn.com/thumbor/mT3JHQtZIyInU8_uGxVH-TCbF50=/0x415:5000x2794/1820x1213/filters:focal(1878x1176:2678x1976):format(webp)/cdn.vox-cdn.com/uploads/chorus_image/image/65171515/1161847141.jpg.0.jpg',
'chelsea_2.jpg')
image = plt.imread('chelsea_2.jpg')
faces = detector.detect_faces(image)
highlight_faces('chelsea_2.jpg', faces)
布拉格斯拉维亚比赛首发 :凯帕、阿兹皮利库塔、路易斯、克里斯滕森、爱默生、康德、巴克利、科瓦西奇、哈扎德、佩德罗、吉鲁德 布拉格斯拉维亚比赛首发 :凯帕、阿兹皮利库埃塔、克里斯滕森、祖玛、爱默生、康德、乔吉诺、科瓦奇、佩德罗、吉鲁德、普利希克
slavia_faces = extract_face_from_image('chelsea_1.jpg')
liverpool_faces = extract_face_from_image('chelsea_2.jpg')
model_scores_starting_xi_slavia = get_model_scores(slavia_faces)
model_scores_starting_xi_liverpool = get_model_scores(liverpool_faces)
``
for idx, face_score_1 in enumerate(model_scores_starting_xi_slavia):
for idy, face_score_2 in enumerate(model_scores_starting_xi_liverpool):
score = cosine(face_score_1, face_score_2)
if score <= 0.4:
# Printing the IDs of faces and score
print(idx, idy, score)
# Displaying each matched pair of faces
plt.imshow(slavia_faces[idx])
plt.show()
plt.imshow(liverpool_faces[idy])
plt.show()
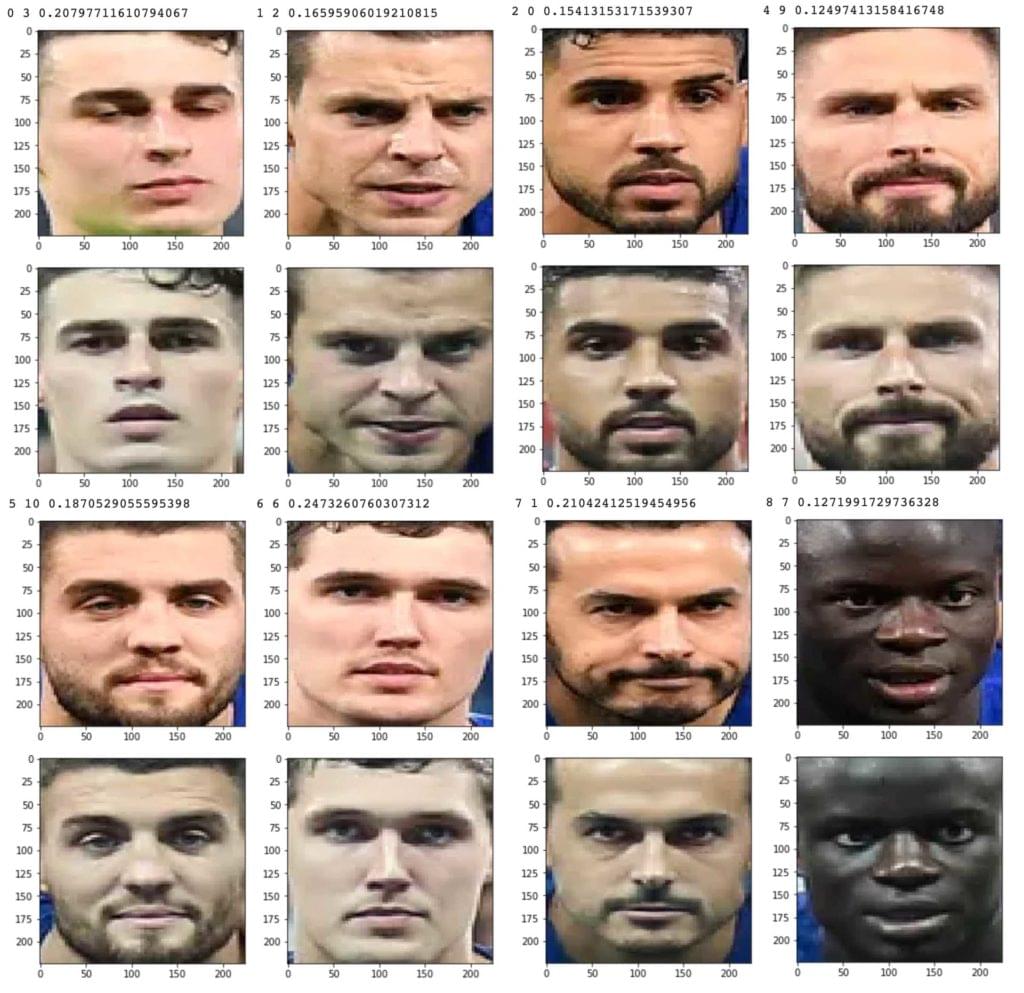
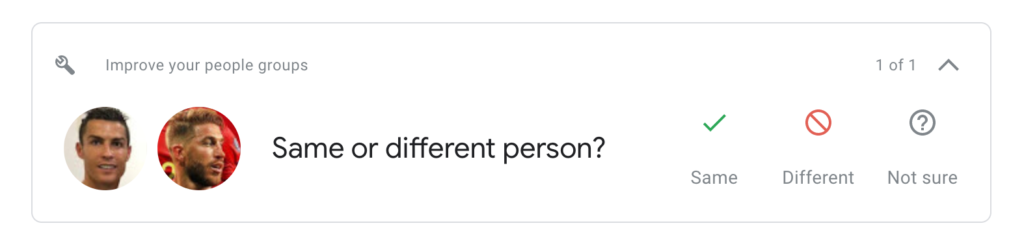